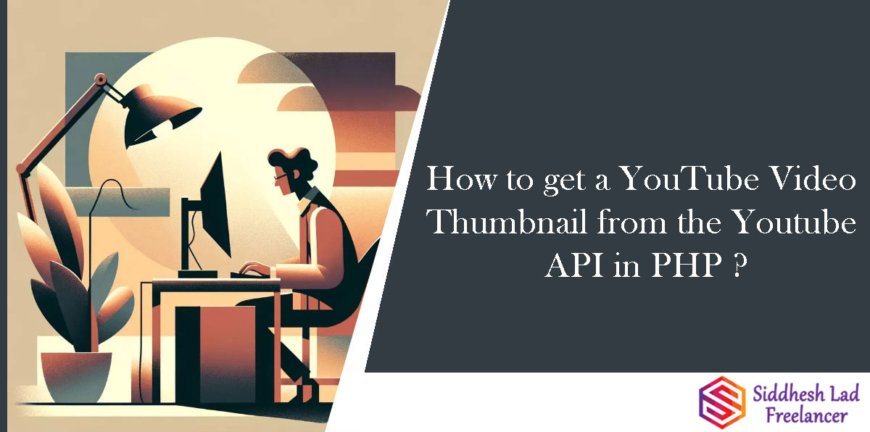
How to get a YouTube Video Thumbnail from the Youtube API in PHP ?
Learn how to easily get a YouTube video thumbnails using the YouTube API in PHP. Step-by-step instructions, code examples, and FAQs to retrieve youtube video thumbnails. YouTube video thumbnails are critical for attracting viewers. A good thumbnail can significantly improve click-through rates, making it essential for content creators and marketers alike. This article will guide you through the process of retrieving YouTube video thumbnails using the YouTube API in PHP.
Article Contents
What is the YouTube API?
The YouTube API is a powerful tool that allows developers to interact with YouTube data programmatically. It offers various functionalities, including searching for videos, retrieving video details, and, of course, fetching video thumbnails. Utilizing the YouTube API can enhance your application by integrating rich YouTube content.
Why Use the YouTube API for Thumbnails?
Using the YouTube API to get video thumbnails provides several advantages:
- Ease of Access: Programmatically retrieve thumbnails without manually searching.
- Dynamic Content: Automatically update thumbnails based on video changes.
- Integration: Seamlessly integrate YouTube content into your applications.
How to Get Started with the YouTube API
Step 1: Set Up Your Google Developer Account
To use the YouTube API, you’ll need a Google Developer account. Here’s how to set it up:
- Visit the Google Developers Console.
- Sign in with your Google account or create a new one.
- Create a new project by clicking on the “Select a project” dropdown and then “New Project.”
- Name your project and click “Create.”
Step 2: Enable the YouTube Data API
Once your project is created, enable the YouTube Data API:
- In the Google Developers Console, navigate to the “Library.”
- Search for “YouTube Data API v3” and select it.
- Click the “Enable” button to activate the API for your project.
Step 3: Obtain API Keys
To make requests to the YouTube API, you’ll need an API key:
- Navigate to the “Credentials” tab on the left sidebar.
- Click “Create Credentials” and choose “API Key.”
- Copy the generated API key for later use.
How to Retrieve YouTube Video Thumbnails Using PHP
Now that you have set up your Google Developer account and obtained your API key, you can start retrieving video thumbnails.
Step 1: Set Up Your PHP Environment
Ensure you have a PHP environment set up. You can use a local server like XAMPP or a web hosting service that supports PHP.
Step 2: Write the PHP Code
Here’s a step-by-step guide to writing the PHP code to get the YouTube video thumbnail.
Step 2.1: Create a PHP File
Create a new PHP file, for example, getThumbnail.php
.
Step 2.2: Write the Code
// Your YouTube API key
$apiKey = 'YOUR_API_KEY';
// The YouTube video ID for which you want to fetch the thumbnail
$videoId = 'VIDEO_ID';// Construct the API URL
$url = "//www.googleapis.com/youtube/v3/videos?id={$videoId}&key={$apiKey}&part=snippet";
// Make the API request
$response = file_get_contents($url);
$data = json_decode($response);
// Check if the response is valid
if (isset($data->items) && count($data->items) > 0) {
// Retrieve the thumbnail URL
$thumbnailUrl = $data->items[0]->snippet->thumbnails->high->url;
echo "Thumbnail URL: " . $thumbnailUrl;
} else {
echo "Error: Video not found or invalid API key.";
}
Step 2.3: Replace Placeholders
Replace YOUR_API_KEY
with your actual API key and VIDEO_ID
with the ID of the YouTube video for which you want to retrieve the thumbnail.
Step 3: Run Your PHP Script
Upload the PHP file to your server or run it locally in your browser. You should see the thumbnail URL printed out if everything is set up correctly.
Understanding the Thumbnail Types
The YouTube API provides different thumbnail resolutions:
- Default: Low resolution (120×90 pixels)
- Medium: Medium resolution (320×180 pixels)
- High: High resolution (480×360 pixels)
- Standard: Standard resolution (640×480 pixels)
- Maxres: Maximum resolution available for the video
You can access these different thumbnail resolutions by changing the object in the code:
$thumbnailUrl = $data->items[0]->snippet->thumbnails->default->url; // Change to 'medium', 'high', etc.
Error Handling in Your PHP Code
When using APIs, it’s crucial to handle errors gracefully. Here’s how to improve your code with error handling:
if (json_last_error() !== JSON_ERROR_NONE) {
echo "Error: Unable to parse response.";
} elseif (!isset($data->items) || count($data->items) === 0) {
echo "Error: Video not found or invalid API key.";
} else {
// Continue with thumbnail retrieval
}
Frequently Asked Questions (FAQs)
How do I find my YouTube video ID?
The video ID is the unique string found in the YouTube URL. For example, in //www.youtube.com/watch?v=VIDEO_ID
, the VIDEO_ID
part is what you need.
Can I get thumbnails for private videos?
No, you cannot retrieve thumbnails for private videos unless you have the necessary permissions.
What if I exceed my YouTube API quota?
If you exceed your quota, you will need to wait for it to reset. Consider optimizing your requests to avoid hitting the limit.
Is there a way to get the thumbnail without the API?
Yes, you can construct the thumbnail URL manually using the video ID. For example: //img.youtube.com/vi/VIDEO_ID/maxresdefault.jpg
How can I cache the thumbnails?
You can implement caching in your application using file storage or databases to save the thumbnail URLs, reducing API calls.
Conclusion
Retrieving YouTube video thumbnails using the YouTube API in PHP is a straightforward process that can significantly enhance your application. With this guide, you now have the tools and knowledge to implement this functionality effectively. Whether for personal projects or larger applications, accessing YouTube content has never been easier.
Ready to get started? Set up your Google Developer account and begin using the YouTube API to enhance your projects today! If you have any questions or need further assistance, feel free to reach out.